Connecting Android Application to IoT-Ignite
In this tutorial, we’re going to use IoT-Ignite SDK for connecting our device to IoT-Ignite cloud.
Firstly create an Android app and set up the required dependencies:
Make sure IotIgniteAgent app is installed on your device. Also, your device must be registered to IoT-Ignite.
You can download and install latest IoT-Ignite Agent app from Google Play Store: Download IoT-Ignite
Add this code block to your application’s MainActivity->onCreate() function.
/** * Sample code for connect to IoT-Ignite cloud. */ try { mIotIgniteManager = new IotIgniteManager.Builder() /* Application Context */ .setContext(getApplicationContext()) /* Connection callback for ignite state. */ .setConnectionListener(new ConnectionCallback() { @Override public void onConnected() { Log.i(TAG,"Ignite connected"); } @Override public void onDisconnected() { Log.i(TAG,"Ignite disconnected"); } }) /* finally build and get instance */ .build(); } catch (UnsupportedVersionException e) { Log.e(TAG,"UnsupportedVersionException : " + e.getMessage());
}
IotIgniteManager is the main entrance for connecting IoT-Ignite. We can generate its instance using
new IotIgniteManager.Builder()
Builder() requires two parameters to generate an instance. These are:
Builder().setContext(Context appContext) : IotIgniteManager using your app context for differentiate you from other apps.
Builder().setConnectionCallback(ConnectionCallback cb) : Connection callback for IoT-Ignite connection.onConnected() function triggers when connection established.
When your app is connected to IoT-Ignite cloud successfully, you should see “Sample IoT-Ignite App: Ignite connected” output in your logcat:
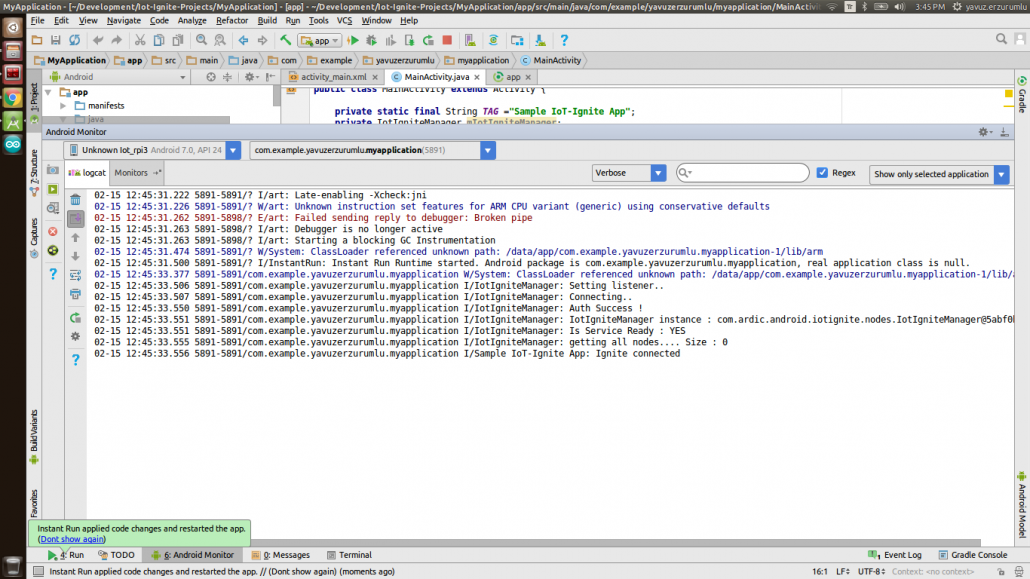
Application Authorization Result
Reasons why an Unsupported Version Exception occurs are:
- IoT-Ignite Agent App is not up to date or your application is using the newest version of IgniteSDK.
Solution: Update your IoT-Ignite Agent application to the latest version. - Your app is using an old version of IgniteSDK but IotIgniteAgent app is the newest version.
Solution: Use the latest version of IgniteSDK.
Complete code for main activity class is:
import android.app.Activity; import android.os.Bundle; import android.util.Log; import com.ardic.android.iotignite.callbacks.ConnectionCallback; import com.ardic.android.iotignite.exceptions.UnsupportedVersionException; import com.ardic.android.iotignite.nodes.IotIgniteManager; public class MainActivity extends Activity { private static final String TAG ="Sample IoT-Ignite App"; private IotIgniteManager mIotIgniteManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); /** * Sample code for connect to IoT-Ignite cloud. */ try { mIotIgniteManager = new IotIgniteManager.Builder() /* Application Context */ .setContext(getApplicationContext()) /* Connection callback for ignite state. */ .setConnectionListener(new ConnectionCallback() { @Override public void onConnected() { Log.i(TAG,"Ignite connected"); } @Override public void onDisconnected() { Log.i(TAG,"Ignite disconnected"); } }) /* finally build and get instance */ .build(); } catch (UnsupportedVersionException e) { Log.e(TAG,"UnsupportedVersionException : " + e.getMessage()); } } }
Happy Codings,